Dart has fully implemented sound null safety to improve code stability and boost developer efficiency. We will explore null safety, its importance, and how to make the most of it in Dart.
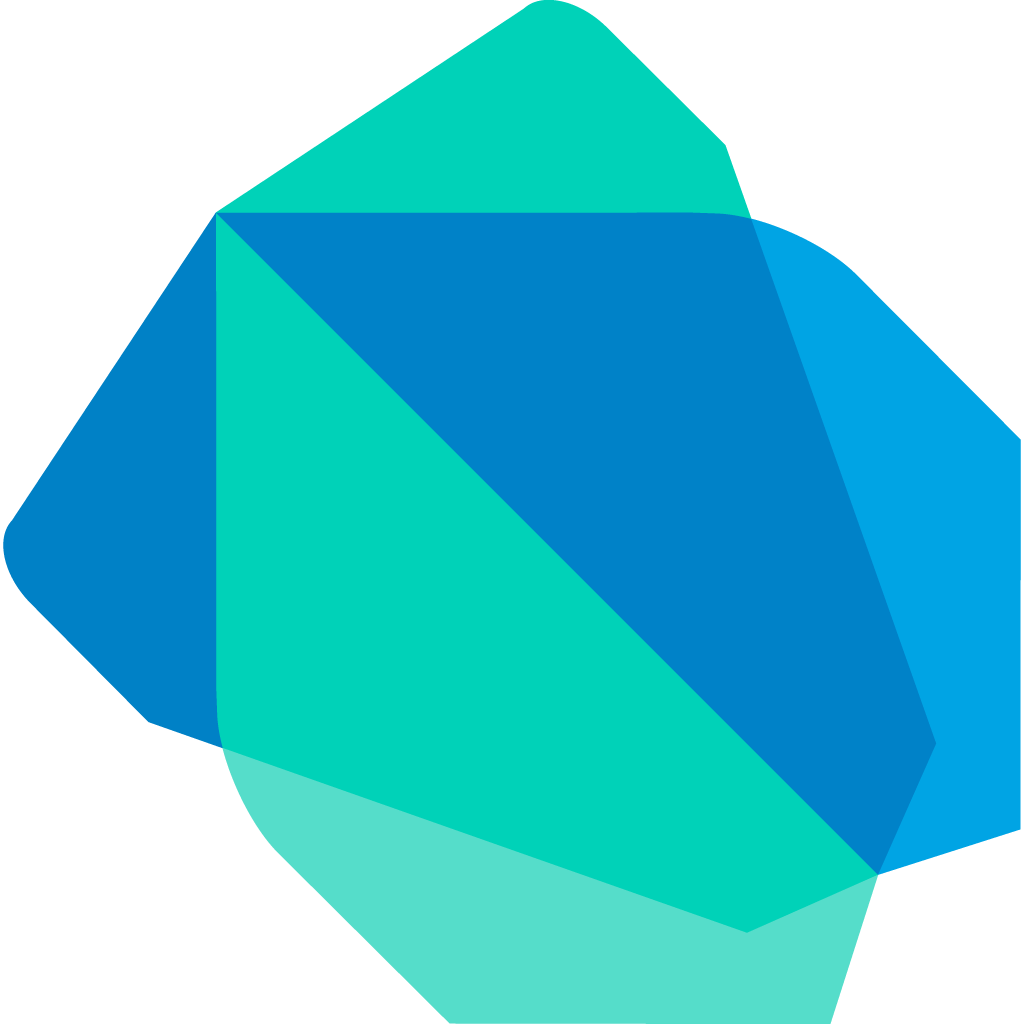
Dart Null Safety
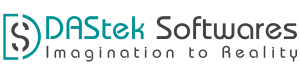
Introduction
Dart has fully implemented sound null safety to improve code stability and boost developer efficiency. We will explore null safety, its importance, and how to make the most of it in Dart.
Nullable vs. Non-Nullable Types:
Non-Nullable Types: For all the dart variables, dart has all the variables that are non-nullable by
default.
String city = âAhmedabadâ; // non-nullable variable
City = null; // not allowed
Nullable: For assigning a null value to any dart variable, you must explicitly declare it as a nullable variable, by adding a question mark (?) at the end of the variable type. String? city = âAhmedabadâ; // nullable String variable city = null; // allowed for this variable type.
Nullable: For assigning a null value to any dart variable, you must explicitly declare it as a nullable variable, by adding a question mark (?) at the end of the variable type. String? city = âAhmedabadâ; // nullable String variable city = null; // allowed for this variable type.
What is Null Safety?
Null safety is a feature in programming languages that makes sure variables donât hold a “null” value
unless you specifically allow it. This helps prevent errors that happen when you try to use something
that doesnât actually exist. In a null-safe system, variables are automatically set to not accept null
values. If you want a variable to be able to be null, you have to clearly mark it as nullable by
a question mark (?) at the end of the variable type.
Why Null Safety is Important
Enhanced Code Safety- Null safety greatly lowers the chances of errors caused by null values, which
often lead to crashes while the program is running. By detecting these issues while coding, Dart helps
create stronger and more reliable code.
Improved Developer Experience- With null safety, developers get instant alerts from the compiler about possible issues with null values. This makes it easier and quicker to fix problems, leading to a smoother development process.
Clearer Code Intent- By clearly marking variables as either nullable or non-nullable, the code explains itself better. Itâs easier to understand what the developer intended, making the code simpler to work with and update.
Improved Developer Experience- With null safety, developers get instant alerts from the compiler about possible issues with null values. This makes it easier and quicker to fix problems, leading to a smoother development process.
Clearer Code Intent- By clearly marking variables as either nullable or non-nullable, the code explains itself better. Itâs easier to understand what the developer intended, making the code simpler to work with and update.
What is Sound Null Safety?
Null safety offers ways to manage null values, but it doesnât always guarantee that non-nullable
variables will never be null. There can be gaps or risky operations that might let null values slip into
non-nullable variables. In these cases, extra checks happen while the program is running, which can
add some processing time and affect performance.
Sound null safety goes a step further by making sure that non-nullable variables canât be assigned null values while coding. The system is strict and catches any possible null assignments before the code runs, which reduces the need for extra checks during runtime. This makes the code safer and faster by ensuring itâs free from null-related errors before it even runs.
Sound null safety goes a step further by making sure that non-nullable variables canât be assigned null values while coding. The system is strict and catches any possible null assignments before the code runs, which reduces the need for extra checks during runtime. This makes the code safer and faster by ensuring itâs free from null-related errors before it even runs.
How to Use Null Safety in Dart
Enabling Null Safety
Null safety is automatically turned on in Dart 2.12 and later versions. To use null safety, make sure
your projectâs settings specify a Dart version that includes it.
environment: SDK: “>=2.12.0 <3.0.0"
environment: SDK: “>=2.12.0 <3.0.0"
Declaring Nullable and Non-Nullable Variables
As previously mentioned, non-nullable variables are declared as usual, while nullable variables are
declared by adding a question mark (?) at the end of the variable type.
String name = âShreeâ; // non-nullable variable
String? city = âBanarasâ; // Nullable variable
name = null;
String name = âShreeâ; // non-nullable variable
String? city = âBanarasâ; // Nullable variable
name = null;
Handling Null Values
In Dart, managing null values means using different tools and features that help handle them safely.
Here are some common ways to work with null values in Dart:
1. The Null-Aware Operator
Dart offers several null-aware operators that make it easier to work with nullable types:
2. The Null-Coalescing Operator (??)
Provide a default value when a nullable variable is null:
String? name;
String newname = name ?? âDefaultâ;
3. The Null Assertion Operator (!) If you’re sure a nullable value isn’t null, you can use the null assertion operator to treat it as if it were non-nullable.
String? name = getName();
int length = name!.length; // throws an error if name is null
4. Null Check If you are using a null check, Dart will automatically nullable type variable to a non-nullable variable. void printLength(String? text) {
if (text != null) {
print(text.length); // text is promoted to non-nullable String
} else {
print(‘Text is null’);
}
}
Here are some common ways to work with null values in Dart:
1. The Null-Aware Operator
Dart offers several null-aware operators that make it easier to work with nullable types:
- Null-aware Assignment (??=)
This operator assigns a value to a variable only if the variable is currently null.String? name; name ??= âGuestâ; print(name); // âGuestâ value will be assigned only if the name is null - Null-aware Access (?.)
This operator lets you call methods or access properties of an object only if the object is not null. If the object is null, it simply returns null without causing an error. String? city = getCity(); Int? length = City?.length; // return length only if it is not null else it will return null - Null-aware Cascade (?..)
Similar to the null-aware access operator but used for chaining multiple operations.
Class {
String? name;
String? Number:
void updateName(String newName) {
this.name = newName;
}
void updateNumber(int newNumber) {
this.name = newNumber;
}
}
Void main {
User? user = User();
user
?..updateName(âRamâ)
..updateNumber(1234567890)
..name = âKrishnaâ;
print(user?.name); // result: Krishna
print(user?.number); // result: 1234567890
}
2. The Null-Coalescing Operator (??)
Provide a default value when a nullable variable is null:
String? name;
String newname = name ?? âDefaultâ;
3. The Null Assertion Operator (!) If you’re sure a nullable value isn’t null, you can use the null assertion operator to treat it as if it were non-nullable.
String? name = getName();
int length = name!.length; // throws an error if name is null
4. Null Check If you are using a null check, Dart will automatically nullable type variable to a non-nullable variable. void printLength(String? text) {
if (text != null) {
print(text.length); // text is promoted to non-nullable String
} else {
print(‘Text is null’);
}
}
Conclusion
Null safety in Dart is a game-changer, making your code more reliable, stable, and easier to maintain. By using null safety, you can avoid common problems caused by null references, leading to a smoother development process and higher-quality software.
By adopting null safety in Dart, you can rest assured that your code is protected from one of the most common and frustrating errors in programming. Happy coding!
By adopting null safety in Dart, you can rest assured that your code is protected from one of the most common and frustrating errors in programming. Happy coding!
Trust and Worth
Our Customers
We are having a diversified portfolio and serving customers in the domains namely Sports Management, Online Laundry System, Matrimonial, US Mortgage, EdTech and so on.
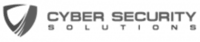
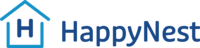


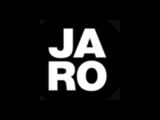
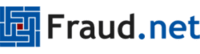
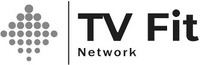
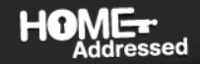
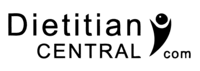

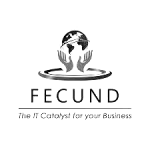
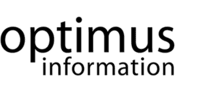

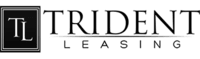
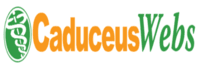
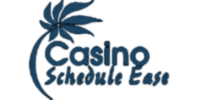
Would you like to start a project with us?
DAStek team would be happy to hear from you and would love to turn your âImaginations to Realityâ.